In this article, we will learn how to implement smooth scrolling to a div in JavaScript. When we click on a link in the header or any link that scrolls the page to a certain section of the same page, we can do that with our HTML code by adding the ID of that section to the href with a #. For example, if we need to target a certain section with id="about"
, then we need to add a link to the anchor tag as #about
. However, this will directly scroll to that position without any scrolling transition. To achieve JavaScript scrolling to a specific div ID with animation, we need to add JavaScript along with this code. So, let’s start implementing this with a simple example. This smooth transition will also work with JavaScript smooth scroll to elements on page load.
Implement Smooth scroll to div ID on click using JavaScript
Let’s start with a simple HTML structure first.
<nav>
<ul>
<li><a href="#section1">Section 1</a></li>
<li><a href="#section2">Section 2</a></li>
<li><a href="#section3">Section 3</a></li>
</ul>
</nav>
<div id="section1" class="section">Section 1 Content</div>
<div id="section2" class="section">Section 2 Content</div>
<div id="section3" class="section">Section 3 Content</div>
In the above code, we have added navigation as the header of the page. Inside the navigation, we have provided some links with the names section1, section2, and section3, and added href anchor tags with # as a prefix. So when the user clicks on the links, it will scroll to the corresponding section ID.
Now add some CSS into this to style this HTML structure as webpage
nav {
position: fixed;
top: 0;
background: #000000;
color: white;
display: flex;
align-items: center;
justify-content: center;
width: 100%;
}
nav ul {
list-style: none;
padding: 10px;
}
nav ul li {
display: inline;
margin-right: 20px;
}
nav ul li a {
color: #000000;
padding: 10px 15px;
background: #ffffff;
border-radius: 5px;
text-decoration: none;
}
.section {
height: 100vh;
padding: 20px;
background: lightgray;
border: 1px solid #ccc;
display: flex;
flex-direction: column;
align-items: center;
justify-content: center;
font-size: 70px;
font-weight: bold;
color: #ffffff;
}
#section1 { background: linear-gradient(to top, #a18cd1 0%, #fbc2eb 100%); }
#section2 { background: linear-gradient(120deg, #89f7fe 0%, #66a6ff 100%);}
#section3 { background-image: linear-gradient(to top, #37ecba 0%, #72afd3 100%);}
In above code we have provided height of each section as 100vh so each section should cover the height of document after adding style it will look like the below screen
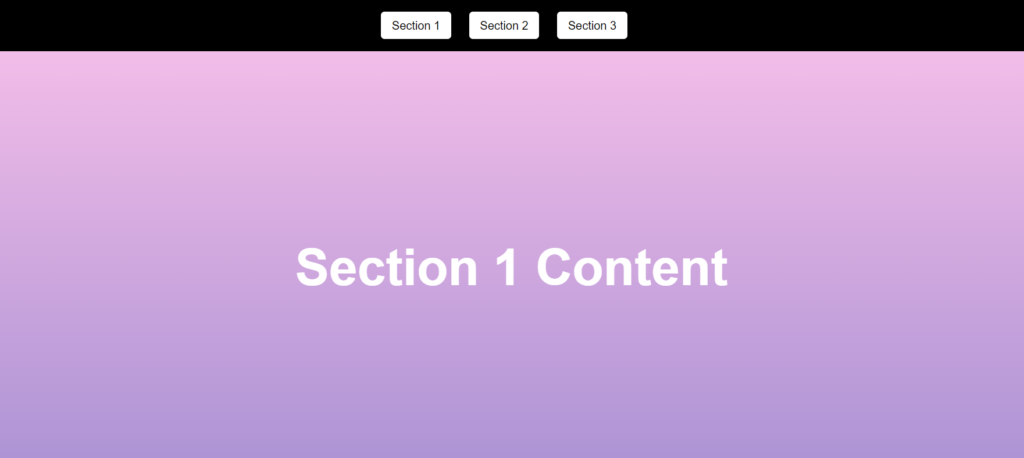
Now when you click on the link the page will diractly targeted to the perticuler section , you will not see any scroll animation , but to achive this animation we need to add below javascrtip
JavaScript smooth scroll with easing function
document.querySelectorAll('a[href^="#"]').forEach(anchor => {
anchor.addEventListener('click', function(e) {
e.preventDefault();
const target = document.querySelector(this.getAttribute('href'));
if (target) {
target.scrollIntoView({
behavior: 'smooth'
});
}
});
});
document.querySelectorAll('a[href^="#"]')
will find the links with the prefix #. For each loop, it will iterate through them and add a click function. When the user clicks on a link, it will smoothly scroll to the corresponding div ID using vanilla JavaScript. Check out the demo below as well.
DEMO
See the Pen Smooth Scroll To Div Element by mohit (@mohit49) on CodePen.