If you are a javascript developer and you do some iteration using javascript for loop and the for compare between two javascript arrays we need to write two different for loops and the we will be able to compare, this all make lots of efforts to a javascript developer and increase the length of the code as well. To get ride of these ittrations and double ittrations there are sevral cool javascript array method are introduced in ES5 and ES6 . so in this blog we are going to understand these methods and how to solve our problems with the help of javascript array methods.
Array Methods In Javascript
- Array.forEach Method
- Array.map Method
- Array.findIndex
- Array.filter Method
- Array.every Method
- Array.some Method
- Array.reduce Method
Now Lets Go through each of the methods listed above and understand with example
Array.forEach Method
The method name itself having meaning for each (each element) so if we have an array this method will help us to itrate with each element of that array , let go with the below exampl of array.
Suppose we have certain names of student which are in array and we want to get perticuler name “sunil” in that array below is the array code
var studentName = ["Raju", "Sunil", "Mayank" , "Neeraj", "Abhishek", "Aditya"]
To itrate each element of the above array we will use array.forEach method where array is the name of the variable i.e studentName. So lets write a code to itrate each element of array.
var studentName = ["Raju", "Sunil", "Mayank" , "Neeraj", "Abhishek", "Aditya"]
studentName.forEach(function(element) {
console.log(element)
})
/* output
Raju
Sunil
Mayank
Neeraj
Abhishek
Aditya
*/
In the console, you will be able to see all the names of student got printed. Now lets check if the element contains student with name sunil, let check the below code.
var studentName = ["Raju", "Sunil", "Mayank" , "Neeraj", "Abhishek", "Aditya"]
studentName.forEach(function(student) {
if(student == "Sunil") {
console.log("Student With Name Sunil is Present")
}
})
/* output
Student With Name Sunil is Present
*/
Note : We need to keep in mind that for each loop will never return a value
As mentioned above in note. For each will never return a value, let understand more with below example
var studentName = ["Raju", "Sunil", "Mayank" , "Neeraj", "Abhishek", "Aditya"]
const studentReturn = studentName.forEach(function(student) {
return student
})
console.log(studentReturn)
/* output
undefined
*/
You will be able to see the above console is undefined , also It does not return any value, even if you explicitly return a value from the callback function
In all the above examples, we have used only the first parameter of the callback function. But the callback function also receives two additional parameters, which are:
- index – the index of the element which is currently being iterated
- array – original array which we’re looping over
var studentName = ["Raju", "Sunil", "Mayank" , "Neeraj", "Abhishek", "Aditya"]
studentName.forEach(function(student,index,array) {
console.log(student, index, array);
})
/* output
Raju 0 (6) ['Raju', 'Sunil', 'Mayank', 'Neeraj', 'Abhishek', 'Aditya']
Sunil 1 (6) ['Raju', 'Sunil', 'Mayank', 'Neeraj', 'Abhishek', 'Aditya']
Mayank 2 (6) ['Raju', 'Sunil', 'Mayank', 'Neeraj', 'Abhishek', 'Aditya']
Neeraj 3 (6) ['Raju', 'Sunil', 'Mayank', 'Neeraj', 'Abhishek', 'Aditya']
Abhishek 4 (6) ['Raju', 'Sunil', 'Mayank', 'Neeraj', 'Abhishek', 'Aditya']
Aditya 5 (6) ['Raju', 'Sunil', 'Mayank', 'Neeraj', 'Abhishek', 'Aditya']
*/
Advantages of using forEach instead of a for loop
- Using a
forEach
loop makes your code shorter and easier to understand - When using a
forEach
loop, we don’t need to keep track of how many elements are available in the array. So it avoids the creation of an extra counter variable. - Using a
forEach
loop makes code easy to debug because there are no extra variables for looping through the array - The
forEach
loop automatically stops when all the elements of the array are finished iterating.
Array.map Method
As above, this method is also used to iterate over an array. The only difference is it will return a new array based on the logic and callback function applied on each element of the array. We need to keep in mind that the array map() method does not modify the original array. Also, in the array map method, the functions are not executable on empty elements of the array.
Array.Map Method Example
Let’s go through a simple example of writing a table of multiples of an array with the number two, which returns a new array as a result.
var serials = [1, 2, 3, 4, 5, 6, 7, 8, 9, 10];
var result = serials.map(function (number) {
return number * 2
})
// output
// [2, 4, 6, 8, 10, 12, 14, 16, 18, 20]
From the above example, we have declared a new variable result
which will store a new array based on what the map
function will return.
If we try to achive above example with for each loop , it will not work , please check the below screen
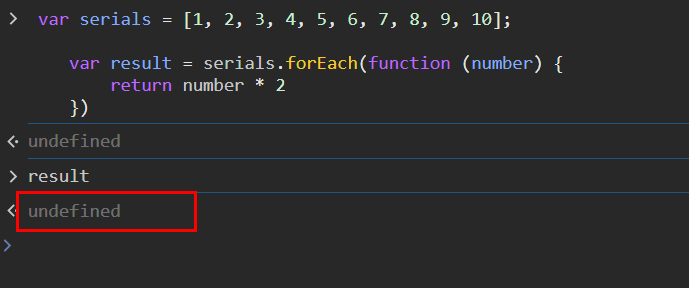
Array.findIndex
Array findIndex method will return the index of the ittrated element with callback function , if the conditions or call back function if false the it will retuen index value as -1 which means the element is not present at any index of the array. Lets go through below example to understand more on it.
var months = ["jan","feb","mar","apr","may"]
function findmonth(element, index, array) {
return (element == "mar");
}
console.log((months.findIndex(findmonth)));
// it will return 2 as result
The above code will return 2 as a result which means the string “Mar” is existing at index 2
Now lets go through one more senario where we pass a string which is not present in the above array
var months = ["jan","feb","mar","apr","may"]
function findmonth(element, index, array) {
return (element == "june");
}
console.log((months.findIndex(findmonth)));
// it will return -1 as result
in the above code june is not present in the array list so it will return result as -1 .
Array.filter() Method
Method name itself provides the method meaning. So it will return a new array as a map function does on the basis os stisying argument conditions it will return a new array.
let go through an example to unserstand more about this method. suppose we need to find people with age more then 18 years who can vote in election.
function canVote(age) {
return age >= 18;
}
function findage() {
let filtered = [24, 33, 16, 40].filter(canVote);
console.log(filtered);
}
findage();
// result [24, 33, 40] new array
So we have a map method also which dose similer things what filter method will do. but then why javascript introduce filter method , lets try to differentiate between map and filter method
Difference Between Map and Filter Method
The map method is used to apply a function on every element of an array, which will return a new array with the same length as the previous array passed to the map method. However, in the case of the filter method, it will return a new array after qualifying the condition passed as an argument, which will filter only those elements that qualify the callback method. In the case of the filter method, the length of the new array may differ from the array initially passed to the filter method.
Array.every Method
With the JavaScript Array every() method, you can assess if all elements in an array satisfy a test defined by a function. It will return true if all elements pass the condition, or false if any fail. This method is practical for validating or checking requirements on array elements.
Let go through an example to understand more about it
// JavaScript code for every() method
function isEven(element, index, array) {
return element % 2 == 0;
}
function func() {
let arr = [56, 92, 18, 88, 12];
// Check for even number
let value = arr.every(isEven);
console.log(value);
}
func();
Output : true
- In this example we Applies the every() method to array arr using the isEven callback function.
- Checks if every element in arr satisfies the condition of being even.
- Returns true if all elements are even; otherwise, returns false.