In this article, we will explore JavaScript DOM manipulation, a key feature of JavaScript that allows developers to modify the Document Object Model (DOM) of a webpage. DOM manipulation enables you to dynamically alter the content and structure of HTML documents. To grasp this concept thoroughly, we’ll start by defining what the DOM is and how it functions.
Understanding The DOM (Document Object Model)
The Document Object Model (DOM) is an interface implemented by browsers to represent web pages as a hierarchical structure of objects. This model allows developers to dynamically update the content and style of HTML documents using JavaScript. With the DOM, you can easily modify attributes, add or remove classes, and make other changes to HTML elements. Understanding the DOM is essential for effective web development and dynamic content manipulation.
Let go though the below HTML structure
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Simple HTML Structure</title>
</head>
<body>
<header>
<h1>Welcome to My Website</h1>
</header>
<main>
<h2>About Us</h2>
<p>This is a simple HTML structure example.</p>
<p>Lorem ipsum dolor sit amet, consectetur adipiscing elit. Nullam scelerisque urna eu felis efficitur, nec dapibus est sollicitudin.</p>
</main>
<footer>
<p>© 2024 My Website</p>
</footer>
</body>
</html>
In the above code structure, we have <html>
, <head>
, <body>
, <header>
, <h1>
, <main>
, and within <main>
, there are <h2>
and <p>
, followed by a <footer>
. Each of these elements is known as a node, and together they form the DOM tree. Let’s render this in the browser and check the screenshot below.
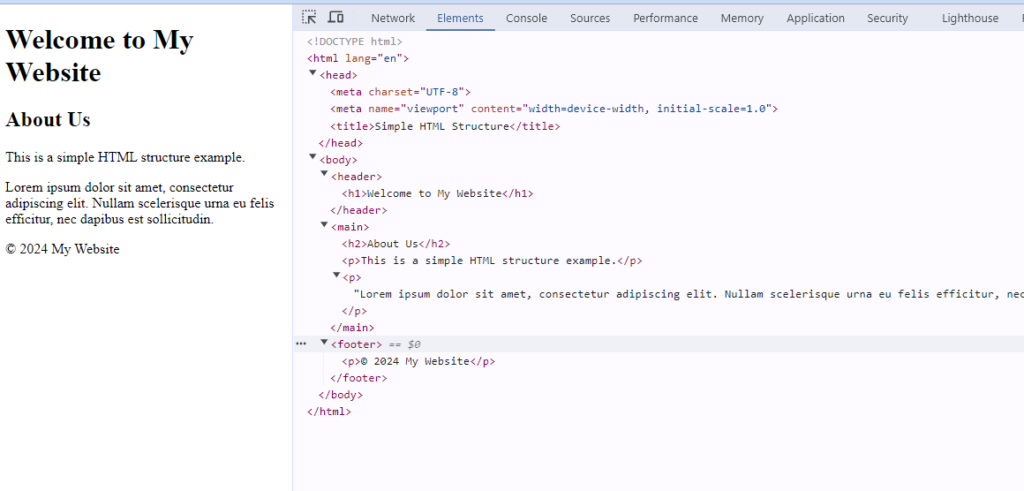
In the above image, you can see on the right side the <!DOCTYPE html>
declaration followed by <html>
, <head>
, and other elements. This collection of elements or nodes is known as the DOM (Document Object Model). Now, let’s learn how to access these DOM nodes.
How To Accessing The DOM Elements
To understand how to perform DOM manipulation in JavaScript, we first need to learn how to access the DOM within our HTML structure. This can be easily achieved by using JavaScript code to interact with the DOM. Some of the most commonly used methods for DOM manipulation are listed below:
Lets suppose we need to access the <header> node as shown in image above, we can simply use the below code for that
const header = element.getElementsByTagName("header");
Now suppose if header have some class name as below
<header class"website-header"></header>
If you want to select and element wich have some class name, so you can access as below
const header = element.querySelector(".website-header");
If we have multiple div with same class and we want to select that then we can update the above method as below, which will select all the elements which contains class website-header
const header = element.querySelectorAll(".website-header");
The above are the most common methods to access the DOM, now lets learn how we can manupulate the dom
Modifying DOM Elements
Once we can able to access the DOM nodes , the developer can easily manupulate the or modify them using various properties and methods
Changing Text Content : If you want to update the content of the element only text you can use below property
header.textContent="Hello World"
Adding the HTML Content : Below is the code if you want to add mode html node inside the element
header.innerHTML = "<div>inner HTML Added </div>"
Changing the style of element : below is the example how we can change the stye of the element
header.style.background = "#00000"
Now Suppose if i want to add new element inside the body then we first need to create and element though javascript code and then need to append inside the body as below
var newElement = document.createElement("div") //you can add any tag you want to create element
// Now we need to select the body and inside that we will append new element
document.querySelector("body").appendChild(newElement);
After Creating element now learn how we can delete the element from dom by using javascript method remove()
newElement.remove()
Now lets learn some points thats needs to be taken care while makeing DOM Manipulation
Things needs to take care while DOM manipulation
1. Minimize Direct DOM Manipulations
- Batch Changes: Make changes to the DOM in batches rather than making frequent updates. This reduces the performance overhead associated with reflows and repaints.
- Use DocumentFragments: When adding multiple elements, use
DocumentFragment
to build up your changes off-screen and then append the fragment to the DOM in one go.
2. Optimize Querying
- Cache Selectors: Store references to frequently accessed elements instead of querying the DOM multiple times.
- Use Efficient Selectors: Prefer using IDs (
#id
) over class names (.class
) or tag names (tag
) for better performance, as IDs are faster to query.
3. Avoid Layout Thrashing
- Minimize Layout Queries: Avoid reading layout properties (like
offsetHeight
,scrollTop
) immediately after writing to the DOM. This can cause layout thrashing, where the browser has to recalculate styles and layouts. - Use RequestAnimationFrame: If you need to measure and update the DOM, use
requestAnimationFrame
to batch layout changes together.
4. Leverage Event Delegation
- Attach Listeners to Parent Elements: Instead of attaching event listeners to many child elements, attach a single event listener to a parent element and use event delegation to handle events from children.
5. Keep Your JavaScript and HTML Separate
- Separation of Concerns: Keep your JavaScript logic separate from HTML. Use JavaScript to manipulate the DOM and maintain your HTML structure as static as possible.
6. Use Modern APIs
classList
for Classes: Useelement.classList
to add, remove, and toggle classes instead of manipulating theclassName
property directly.dataset
for Custom Attributes: Usedataset
to handle custom data attributes.
7. Avoid Inline Styles
- Use CSS Classes: Instead of setting styles directly via JavaScript, use CSS classes to manage styling. This keeps your styling rules in one place and makes your code more maintainable.
8. Optimize for Accessibility
- Use Semantic HTML: Ensure your DOM manipulation does not undermine the accessibility of your application. Use semantic HTML elements and ARIA roles where appropriate.
9. Test Across Browsers
- Cross-Browser Compatibility: Test your DOM manipulations across different browsers to ensure consistent behavior and appearance.
10. Document Your Code
- Comment and Document: Provide clear comments and documentation for your DOM manipulation code. This helps others (and future you) understand the purpose and function of your code.
11. Performance Monitoring
- Profile and Monitor: Use browser developer tools to profile and monitor the performance of your DOM manipulations. Look out for slow operations and optimize them as needed.
Manipulate DOM in SPA using mutation observer
You can also manipulate DOM in single page application (SPA ) below is the link for that