In a React application, normal vanilla JavaScript may not work for some concepts. For example, if I want to toggle a class on a button click or add and remove classes, I might need to show a popup on the website. This can be easily achieved in vanilla JavaScript using the element.classList.toggle("newClass")
method, allowing us to add custom classes. However, in React, we need to use React hooks to achieve this functionality, as it is the recommended and preferred approach in React applications. React for several reasons, primarily related to React design principles and state management.
React’s Virtual DOM: React operates on a virtual DOM, which is an abstraction of the actual DOM. When you manipulate the DOM directly using methods like classList.toggle()
, you bypass React’s reconciliation process. This can lead to inconsistencies between the virtual DOM and the actual DOM, resulting in unexpected behavior and rendering issues.
State Management: React encourages managing UI state through its state management system. Instead of directly toggling classes, React developers typically use state variables to determine which classes should be applied. This approach ensures that the UI reflects the current application state accurately. For example, you would set a state variable to track whether a menu is open or closed and conditionally apply classes based on that state in the render()
method,
Performance: Direct DOM manipulation can lead to performance issues, especially in larger applications. React diffing algorithm is optimized for performance, and using state to manage classes allows React to efficiently update only the parts of the DOM that have changed, rather than re-rendering entire components.
React Toggle class using react useState() hook
Step 1: Import useState
First, you need to import the useState
hook from React:
import React, { useState } from 'react';
Step 2: Create a Functional Component
import React from 'react';
import { useState } from 'react';
import './style.css';
export default function App() {
const [active, setActive] = useState(false);
return (
<div>
<center>
<h1 className={active ? "active" : "in-active"}>Understanding useState with Previus value</h1>
<button onClick={() => setActive(!active)}>Active Button</button>
</center>
</div>
);
}
In the above code, we have imported the React hook useState()
and added the style file ./style.css
. The basic concept of this code is to toggle a class on a button click by utilizing React state management.
Now lets go through one more example by creating a count on button click, so once i click on button it will increase the value of a counter by one.
React Increment value by one on button click by using useState hook
We will set a variable which have a value 1 from start and when we click on a button it will add or plus 1 to the existing value, so if the initial value is one then on button click it will add one more to the existing value and becomes two, below is the code example
import React from 'react';
import { useState } from 'react';
import './style.css';
export default function App() {
const startVal = 0;
const [initialVal, changeVal] = useState(startVal);
return (
<div>
<center>
<h1>Understanding useState with Previus value</h1>
<button onClick={() => changeVal(initialVal + 1)}>Increase</button>
</center>
<h1>
<center>{initialVal}</center>
</h1>
</div>
);
}
In above code we are changing the state based on previous value like if my previous value is one , then on button click we are adding one more value and making it two, so in below code lets check how we can get previous value
Let suppose now on another button click i want to decrease the value now
import React from 'react';
import { useState } from 'react';
import './style.css';
export default function App() {
const startVal = 0;
const [initialVal, changeVal] = useState(startVal);
// not decrease more then below 0
const mindecrese = () => {
changeVal((prevVal) => {
if (prevVal <= 0) {
return prevVal;
} else {
return prevVal - 1;
}
});
};
return (
<div>
<center>
<h1>Understanding useState with Previus value</h1>
<button onClick={() => changeVal(initialVal + 1)}>Increase</button>
<button onClick={mindecrese}>decrease</button>
</center>
<h1>
<center>{initialVal}</center>
</h1>
</div>
);
}
In above code to check previous value we have written a below function
changeVal((prevVal) => {
if (prevVal <= 0) {
return prevVal;
} else {
return prevVal - 1;
}
});
when we are decreasing we are checking if previous value is already 0 if not the it will decrease one more value from the previous value
We have provided the working stack blitz link where we have added all the above working code for react useState() concepts please check the below url
https://stackblitz.com/~/github.com/mohit49/react-useState-with-previus-state?file=src/App.js
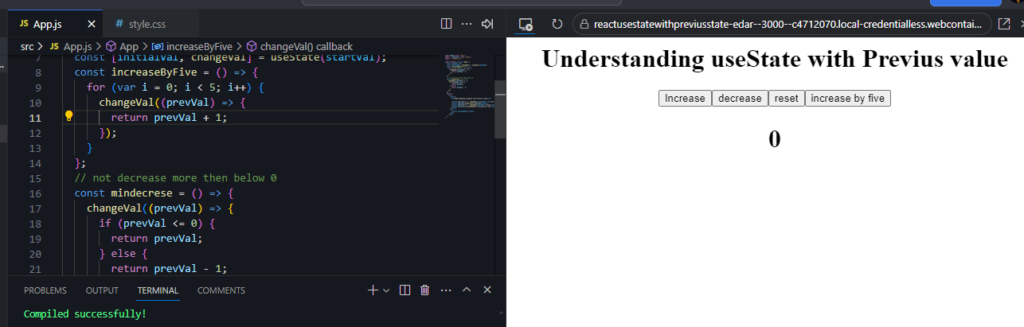